DETECT BROWSER PLUGINS WITH JAVASCRIPT
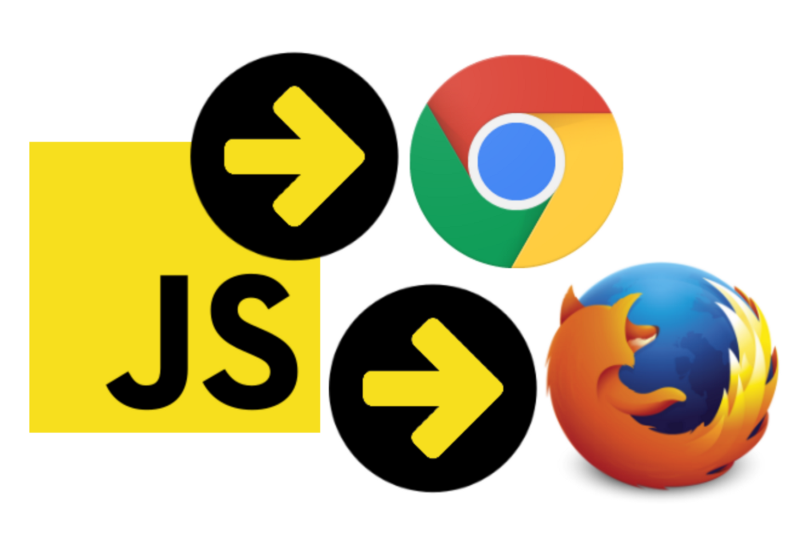
While working on a project a few days ago, I needed to know if the user had Flash or QuickTime installed. After a little bit of searching I found a few solutions online.
This gave me the idea to create a script that checks for plug-ins installed in a browser. I know there are a lot of scripts of this nature online, but if you have the time, check out mine as well, you might find it useful.
The basic idea was to be able to do something like this:if(plugin.available) {do this;} else {do that;}
In standard compliant browsers it’s pretty easy to check if a plug-in is installed. You use the navigator object and check the mimeTypes property.if(navigator.mimeTypes && window.console && window.console.log){ for(var p in navigator.mimeTypes){ window.console.log(navigator.mimeTypes[p]); } }
Let me explain the code above a bit. We are doing what is called object detection or feature detection. We are basically testing to see if the browser supports a certain method or property. In this case we are first testing to see if the browser supports the mimeTypes property of the navigator object.
This property returns the mimeTypes that the browser in question supports. Then we test to see if our browser supports the console object and it’s log method.
If all those conditions are met, then we loop through all of the items in the navigator.mimeTypes property and log each one in the console.
We will get a list of objects that represent our installed plug-ins. We can log the type of each item to see the mimeType.if(navigator.mimeTypes && window.console && window.console.log){ for(var p in navigator.mimeTypes){ window.console.log(navigator.mimeTypes[p].type); } }
You will get something like this:application/googletalk application/vnd.gtpo3d.auto application/x-CorelXARA application/vnd.xara image/vnd.djvu image/x.djvu image/x-djvu image/x-iw44 image/x-dejavu image/djvu
The actual content that will be logged in the console depends on what plug-ins you have installed, so your results may be different from mine.
The problem is that in IE, the navigator.mimeTypes gives us no info about the plug-ins installed(Thanks Microsoft). If we want to check for a plug-in in IE we have to use the ActiveX control. Again, we have to do a little bit of JavaScript feature detection. Since IE is the only browser that recognizes ActiveX controls, if we try to create a new ActiveXObject without checking for support first, standard compliant browsers will throw errors.
We first check to see if the browser knows what an ActiveXObject is:if (typeof ActiveXObject != 'undefined') { try { new ActiveXObject('ActiveXObjectName'); } catch (err) {} }
Then we use a try{} catch(){} statement, because if we did something like:if(typeof new ActiveXObject('ActiveXObjectName') != 'undefined'){....}
IE would throw an error if we passed in an argument it did not recognize.
So let’s get started on our code. First we need to create an object that we will use to store information on our plug-ins in:var installedPlugins = {};
The next step is to add properties to that object. A very simple one to add is support for Java:var installedPlugins = {'java': navigator.javaEnabled()};
If Java is installed, installedPlugins.java will return true , else it will return false.
The next step is to create a self invoking anonymous function. This is done to protect the global scope.(function(){....})()
Now we need to create an object that holds the plug-ins we want to check for. There are literally hundreds of browser plug-ins on the out there, I have made this script to test only for some of the most popular.var plugins = {'flash': { 'mimeType': ['application/x-shockwave-flash'], 'ActiveX': ['ShockwaveFlash.ShockwaveFlash', 'ShockwaveFlash.ShockwaveFlash.3', 'ShockwaveFlash.ShockwaveFlash.4', 'ShockwaveFlash.ShockwaveFlash.5', 'ShockwaveFlash.ShockwaveFlash.6', 'ShockwaveFlash.ShockwaveFlash.7'] }, 'shockwave-director': { 'mimeType': ['application/x-director'], 'ActiveX': ['SWCtl.SWCtl', 'SWCt1.SWCt1.7', 'SWCt1.SWCt1.8', 'SWCt1.SWCt1.9', 'ShockwaveFlash.ShockwaveFlash.1'] }, .......... 'quicktime': { 'mimeType': ['video/quicktime'], 'ActiveX': ['QuickTime.QuickTime', 'QuickTimeCheckObject.QuickTimeCheck.1', 'QuickTime.QuickTime.4'] }, 'windows-media-player': { 'mimeType': ['application/x-mplayer2'], 'ActiveX': ['WMPlayer.OCX', 'MediaPlayer.MediaPlayer.1'] } };
Our object contains a list of plug-in names. Each plug-in has two properties: mimeType and ActiveX. mimeType is used for checking in standard compliant browsers and ActiveX is used for IE.
Now that we have our plug-ins object set up, we need to create the function that will check for support://check support for a plugin function checkSupport(p){ var support = false; //for standard compliant browsers if (navigator.mimeTypes) { for (var i = 0; i < p['mimeType'].length; i++) { if (navigator.mimeTypes[p['mimeType'][i]] && navigator.mimeTypes[p['mimeType'][i]].enabledPlugin) { support = true; } } } //for IE if (typeof ActiveXObject != 'undefined') { for (var i = 0; i < p['ActiveX'].length; i++) { try {new ActiveXObject(p['ActiveX'][i]); support = true; } catch (err) {} } } return support; }
We start by creating a function called checkSupport(). This function accepts one parameter, or argument and that is the plug-in we are testing for. The function returns true if it finds that a certain mimeType or ActiveX control is supported by the browser and false otherwise.
Now that we have our function set up, we need to loop over the plug-ins in our plugins object and test to see if each one is installed or not.//we loop through all the plugins for (plugin in plugins) { //if we find one that's installed... if (checkSupport(plugins[plugin])) { //we add a property that matches that plugin's name to our "installedPlugins" object and set it's value to "true" installedPlugins[plugin] = true; //and add a class to the html element, for styling purposes document.documentElement.className += ' ' + plugin; } else { installedPlugins[plugin] = false; } }
To test if a browser plug-in is installed you need to check the installedPlugins object. Like this:if(installedPlugins.flash){....} else if(installedPlugins.quicktime){....} else if(installedPlugins.windows-media-player){....}
A class containing the plug-in’s name is added to the html element, just in case you need to style the page differently if a plug-in is installed/missing.<html class="3dmlw djvu google-talk flash shockwave-director quicktime windows-media-player pdf vlc xara silverlight">...</html>
Please note that this script only checks for a few plug-ins, those which are most used. If you wish to check for another plug-in you will need to add the necessary info to the plugins object.
The script currently checks for:
Java
3D Markup Language for Web
DjVu
Flash
Google Talk
Acrobat Reader
QuickTime
RealPlayer
SVG Viewer
Shockwave
Silverlight
Skype
VLC
Windows Media Player
Xara
If you find any problems with the code or have any suggestions on how to improve it, please leave a comment.